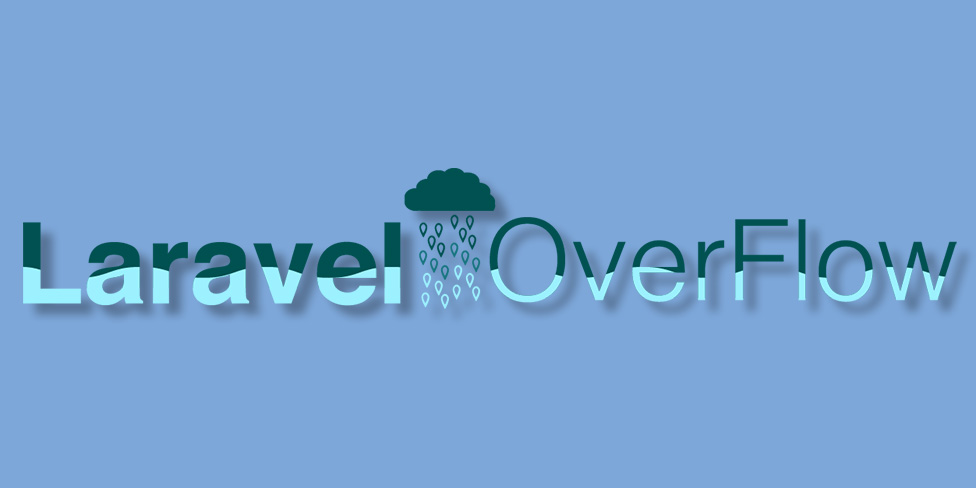
Building Laravel Overflow
Logan Craft • January 20, 2020
projectBuilding Laravel Overflow
Last week I released a package entitled Laravel Overflow on GitHub! In overflow, you can extend your Laravel form requests with the OverflowFormRequest. This allows you to catch extra parameters passed in with a HTTP request that are not defined in a database column within the Eloquent model.
The idea for Laravel Overflow came about because I was needing to create a form builder, and I considering how I might store the configuration of the form within the database. Of course you need to store the form in JSON format. However, I thought to myself, wouldn’t be cool if could take off the different fields and store them in one column if they were not defined on the database? So I composed some syntax for my concept below:
TestModel::create($request->allWithOverflow())
The line of code above was the fundamental concept behind creating the package! At first, I thought my concept would be awesome because I could just use a macro since the request model includes the Macroable trait. However, I would have to pass in a model with some syntax below:
TestModel::create($request->allWithOverflow(new TestModel())
I elected not to go with the option above, but eventually, I might come around to the idea because it would be nice to use the generic Illuminate HTTP Request.
I ended up extending a Form Request for the simplistic api and syntax I wanted. Although this requires a little more setup than I would like, I believe it creates a separation of concerns. Below is a guide for getting Laravel Overflow up and running in your project.
First you will need to install Laravel Overflow in your Laravel app by running the composer command below:
composer require craftlogan/laravel-overflow
Once Overflow is installed, you must use custom form request objects to extend the OverflowFormRequest.
An example of using a custom form request in a controller method would look similar to the code below:
public function store(CustomStoreRequest $request)
{
$testmodel = TestModel::create($request->allWithOverflow());
return $testmodel;
}
Your CustomStoreRequest class should Extend the OverflowFormRequest
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
use CraftLogan\LaravelOverflow\Requests\OverflowFormRequest
class CustomStoreRequest extends OverflowFormRequest
{
public function __construct()
{
parent::__construct(new TestModel); // Your Eloquent Model
}
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return true;
}
/**
* Get the validation rules that apply to the request.
*
* @return array
*/
public function rules()
{
return [];
}
}
That is basically all that is needed with the exception of adding a properties column to the migration of the eloquent class where you want to store the overflow.
$table->json('properties');
If you have some thoughts on this package, please open a GitHub issue, and I would be happy to converse with you! As always, I am very grateful to any contributors. Have a wonderful day and God Bless!
- Logan Craft